Change points#
Joseph Hall (October 2019)
This notebook demonstrates the use of the ChangePoints
kernel, which can be used to describe one-dimensional functions that contain a number of change-points, or regime changes. The kernel makes use of sigmoids (
where
[1]:
import gpflow
import numpy as np
import matplotlib.pyplot as plt
np.random.seed(123) # for reproducibility of this notebook
plt.style.use("ggplot")
%matplotlib inline
def plotkernelsample(k, ax, xmin=-3, xmax=3, title=None):
xx = np.linspace(xmin, xmax, 100)[:, None]
ax.plot(xx, np.random.multivariate_normal(np.zeros(100), k(xx), 3).T)
ax.set_title(title)
2022-05-10 10:48:08.769177: W tensorflow/stream_executor/platform/default/dso_loader.cc:64] Could not load dynamic library 'libcudart.so.11.0'; dlerror: libcudart.so.11.0: cannot open shared object file: No such file or directory
2022-05-10 10:48:08.769201: I tensorflow/stream_executor/cuda/cudart_stub.cc:29] Ignore above cudart dlerror if you do not have a GPU set up on your machine.
We demonstrate the use of the kernel by drawing a number of samples from different parameterizations. Firstly, a simple single change-point between two kernels of differing lengthscales.
[2]:
np.random.seed(1)
base_k1 = gpflow.kernels.Matern32(lengthscales=0.2)
base_k2 = gpflow.kernels.Matern32(lengthscales=2.0)
k = gpflow.kernels.ChangePoints([base_k1, base_k2], [0.0], steepness=5.0)
f, ax = plt.subplots(1, 1, figsize=(10, 3))
plotkernelsample(k, ax)
2022-05-10 10:48:11.940242: W tensorflow/stream_executor/platform/default/dso_loader.cc:64] Could not load dynamic library 'libcuda.so.1'; dlerror: libcuda.so.1: cannot open shared object file: No such file or directory
2022-05-10 10:48:11.940273: W tensorflow/stream_executor/cuda/cuda_driver.cc:269] failed call to cuInit: UNKNOWN ERROR (303)
2022-05-10 10:48:11.940290: I tensorflow/stream_executor/cuda/cuda_diagnostics.cc:156] kernel driver does not appear to be running on this host (49c966262641): /proc/driver/nvidia/version does not exist
2022-05-10 10:48:11.940542: I tensorflow/core/platform/cpu_feature_guard.cc:151] This TensorFlow binary is optimized with oneAPI Deep Neural Network Library (oneDNN) to use the following CPU instructions in performance-critical operations: AVX2 AVX512F FMA
To enable them in other operations, rebuild TensorFlow with the appropriate compiler flags.
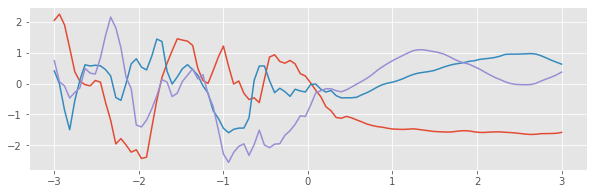
Secondly, an implementation of a “change window” in which we change from one kernel to another, then back to the original.
[3]:
np.random.seed(3)
base_k1 = gpflow.kernels.Matern32(lengthscales=0.3)
base_k2 = gpflow.kernels.Constant()
k = gpflow.kernels.ChangePoints([base_k1, base_k2, base_k1], locations=[-1, 1], steepness=10.0)
f, ax = plt.subplots(1, 1, figsize=(10, 3))
plotkernelsample(k, ax)
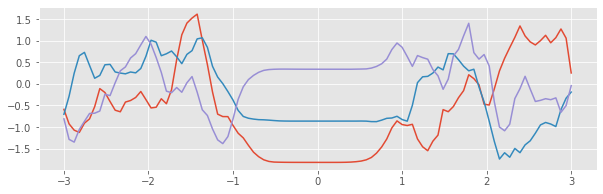
And finally, allowing different change-points to occur more or less abruptly by defining different steepness parameters.
[4]:
np.random.seed(2)
base_k1 = gpflow.kernels.Matern32(lengthscales=0.3)
base_k2 = gpflow.kernels.Constant()
k = gpflow.kernels.ChangePoints(
[base_k1, base_k2, base_k1], locations=[-1, 1], steepness=[5.0, 50.0]
)
f, ax = plt.subplots(1, 1, figsize=(10, 3))
plotkernelsample(k, ax)
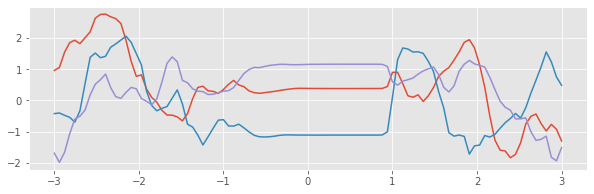